Keras is one of the most popular high-level deep learning libraries in Python. Built on top of TensorFlow, it provides an intuitive interface for building and training machine learning models. If you’re diving into deep learning or data science, you’ll likely want to run Keras within a Jupyter Notebook environment to create and test your models interactively. This guide will walk you through how to install Keras on Jupyter Notebook, covering installation on Windows, macOS, and VS Code. Additionally, we’ll explore how to install Keras alongside TensorFlow to ensure you have everything you need to start building your deep learning models.
What is Keras?
Keras is an open-source deep learning library written in Python that runs on top of other libraries, primarily TensorFlow. It was designed to simplify the process of creating deep learning models by providing high-level abstractions for common tasks such as defining layers, loss functions, and optimizers.
Here are some key features that make Keras popular:
- Easy to use: Its simple API allows you to quickly build and experiment with neural networks.
- Extensible: Keras allows you to define complex models with custom layers and loss functions.
- Fast prototyping: You can rapidly test and modify models, which is ideal for research and experimentation.
- Integration with TensorFlow: Since TensorFlow is the backend for Keras, you get access to powerful deep learning tools and optimized performance.
Now, let’s explore how to install Keras on Jupyter Notebook in various environments.
Pre-requisites for Install Keras on Jupyter Notebook
Before installing Keras, it’s important to ensure that you have Python installed on your system. You will also need pip, Python’s package installer, which you can use to install Keras and its dependencies.
If you don’t have Python installed, download it from the official Python website. During installation, make sure to check the box that says “Add Python to PATH”.
Next, install Jupyter Notebook if you don’t already have it. You can do this by running:
pip install notebook
Once Python and Jupyter Notebook are ready, you can begin installing Keras.
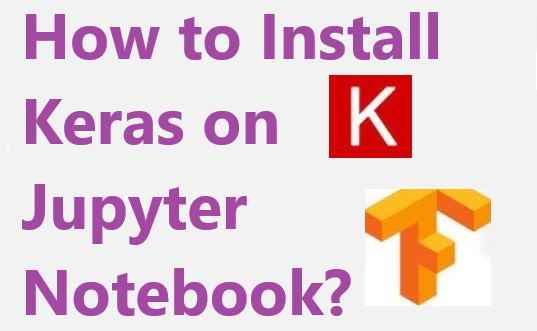
How to Install Keras on Jupyter Notebook in Windows
Installing Keras on Windows is a straightforward process. Here’s how you can do it:
Step 1: Install TensorFlow
Keras is bundled with TensorFlow, which is its backend, so you need to install TensorFlow first. In Jupyter Notebook, you can install TensorFlow by running the following command:
!pip install tensorflow
TensorFlow is a large library, so the installation may take some time. Once TensorFlow is installed, Keras will automatically be available since it is part of TensorFlow.
Step 2: Verify Installation
Once installation is complete, you can verify that Keras is installed properly by running this code in a Jupyter Notebook cell:
import tensorflow as tf
from tensorflow import keras
print(tf.__version__) # Print TensorFlow version to verify installation
If everything is set up correctly, it will print the version of TensorFlow installed, indicating that Keras is ready to use.
How to Install Keras on Jupyter Notebook in macOS
The installation process on macOS is quite similar to Windows, but there are a few system-specific things to keep in mind. Here’s how to install Keras on Jupyter Notebook on macOS:
Step 1: Install Homebrew (Optional)
If you don’t have Homebrew (a package manager for macOS), you can install it by running the following command in your terminal:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
While Homebrew is optional for installing Python and Jupyter, it can make things easier in the future for managing packages.
Step 2: Install TensorFlow (Which Includes Keras)
To install TensorFlow and Keras on macOS, open the terminal and run:
pip3 install tensorflow
This will install the latest version of TensorFlow and Keras.
Step 3: Launch Jupyter Notebook
Once TensorFlow is installed, you can launch Jupyter Notebook by typing the following command in the terminal:
jupyter notebook
This will open Jupyter Notebook in your default web browser. You can now run the code to verify that Keras is installed:
import tensorflow as tf
from tensorflow import keras
print(tf.__version__) # Check TensorFlow version
How to Install Keras on Jupyter Notebook in VS Code
If you prefer working in Visual Studio Code (VS Code), you can install Keras within a Jupyter Notebook using VS Code’s integrated terminal. Here’s how to do it:
Step 1: Install Python Extension for VS Code
If you don’t already have it, install the Python extension for VS Code. It provides support for Python scripts and Jupyter Notebooks.
Step 2: Create a Virtual Environment (Optional but Recommended)
It’s a good practice to use a virtual environment for your projects to avoid conflicts between different package versions. You can create a virtual environment by running:
python -m venv myenv
Activate the virtual environment:
- On Windows:
myenv\Scripts\activate
- On macOS/Linux:
source myenv/bin/activate
Step 3: Install TensorFlow and Keras
Once the environment is activated, install TensorFlow by running:
pip install tensorflow
This will install both TensorFlow and Keras since Keras is part of TensorFlow.
Step 4: Open Jupyter Notebook in VS Code
You can now open VS Code and launch Jupyter Notebook inside it. Create a new notebook, and in the first cell, run:
import tensorflow as tf
from tensorflow import keras
print(tf.__version__) # Check TensorFlow version
This will confirm that Keras is installed and integrated into your VS Code setup.
How to Install Keras on Jupyter Notebook TensorFlow
When you install TensorFlow, Keras is already included as part of the package. However, in some cases, you may want to install a specific version of Keras or use a standalone installation of Keras.
Step 1: Install TensorFlow (Which Includes Keras)
To install the latest version of TensorFlow (which includes Keras), simply run:
pip install tensorflow
This command will install both TensorFlow and Keras. The installation process might take some time, depending on your internet speed and system specifications.
Step 2: Verify Installation
Once the installation is complete, verify that Keras is available by running this code in a Jupyter Notebook cell:
import tensorflow as tf
from tensorflow import keras
print(tf.__version__) # Verify TensorFlow version
If everything is set up correctly, the TensorFlow version will be printed, and you’re ready to start using Keras for building deep learning models.
Troubleshooting Common Installation Issues
While the installation process is generally straightforward, you may run into a few issues. Here are some common problems and how to resolve them:
Issue 1: “ModuleNotFoundError: No module named ‘tensorflow'”
This error usually occurs when TensorFlow is not installed properly. You can fix this by running:
pip install tensorflow
Ensure that you’re using the correct version of Python and pip.
Issue 2: “Keras is not recognized”
If you encounter this issue, it may be because Keras was not installed correctly with TensorFlow. To resolve this, simply install the latest version of TensorFlow using:
pip install --upgrade tensorflow
Issue 3: Jupyter Notebook Not Recognizing TensorFlow
Sometimes, Jupyter Notebook might not recognize installed libraries. You can resolve this by installing the ipykernel
package and linking the environment to Jupyter:
pip install ipykernel
python -m ipykernel install --user
This should allow you to use TensorFlow and Keras inside your Jupyter Notebook.
Conclusion
In this guide, we’ve covered everything you need to know about how to install Keras on Jupyter Notebook, from installing TensorFlow to verifying that Keras is working correctly. Whether you’re using Windows, macOS, VS Code, or integrating TensorFlow with Jupyter Notebook, you now have the tools to start building deep learning models.
By following these steps, you can easily set up Keras in Jupyter Notebook and begin experimenting with machine learning. From installing TensorFlow to running the first few lines of code, you now have the knowledge to work with one of the most powerful libraries in the Python ecosystem. Happy coding, and enjoy your deep learning journey!
Read Also : How to Install NumPy on Jupyter Notebook?